Making payment request with Vendy using the checkout widget
The Vendy checkout widget handles creating a transaction in your web application. To use, you should have your api key which is found in the business object. Check out Get Businesses to get your business and api key or Create a Business to get started.
About the Widget:
The Vendy checkout widget is a lightweight application that embeds an iframe which fills the screen with a light blue overlay with little white "X" at the top right corner of the screen that closes the widget but doesn’t cancel the transaction once it has begun processing and a small responsive modal that displays the progress and state of the transaction in stages. The progress of a transaction has four stages:
-
In the first stage, the request is processed by Vendy and sent to the customer's phone number
-
Next, the customer authorises the request
-
After successful authorisation, The customer is debited using whatever payment method is chosen
-
Payment is completed and transaction ends
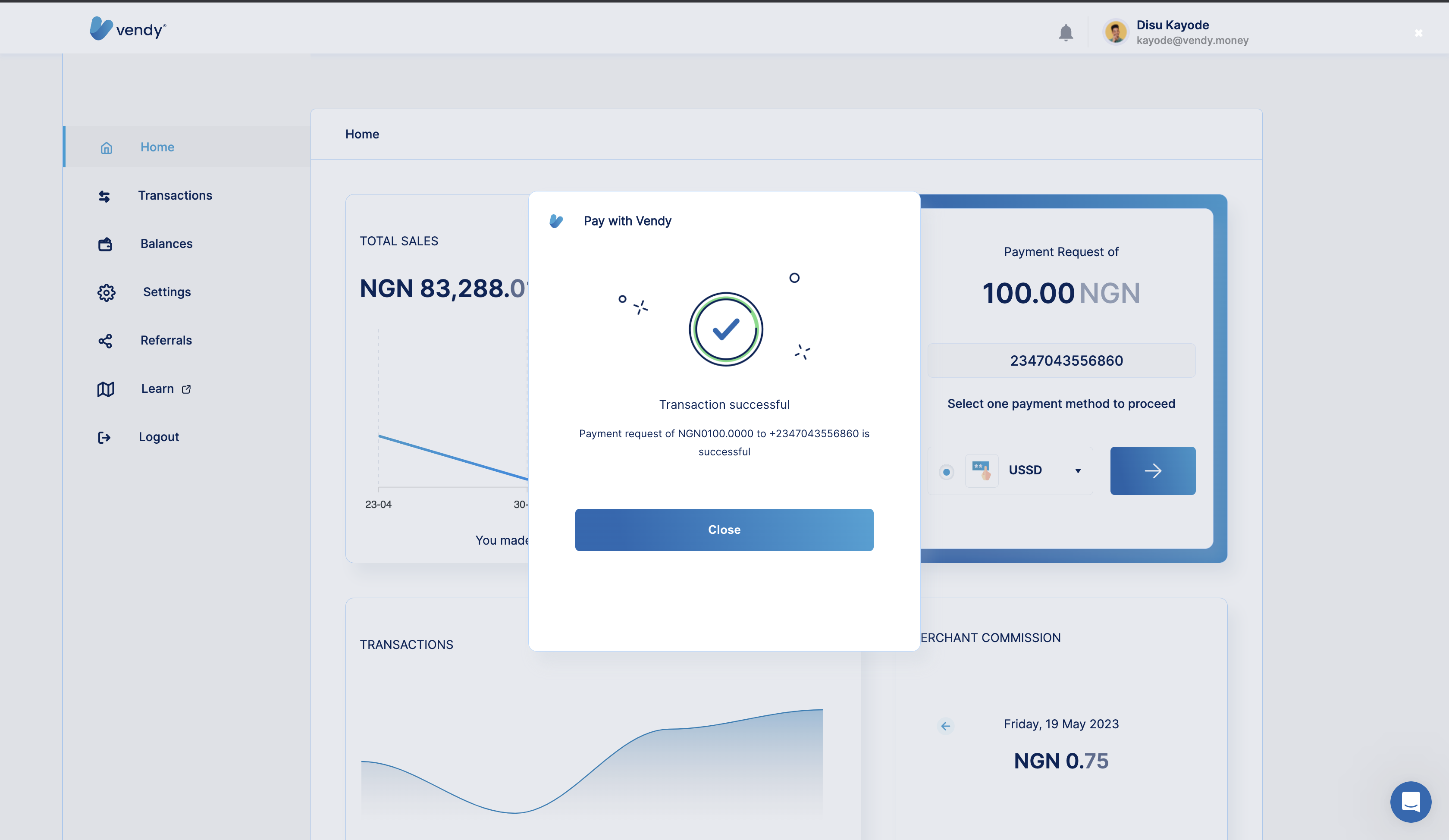
How to use the widget:
-
Import via the script tag into the application.
<script src="https://checkout.vendy.money/v1/inline.js" type="text/javascript" charset="utf-8"></script>
-
Create a one time object of VendyPay that can be used to initialise transactions throughout the application.
const vendyPay = new VendyPay();
-
Call the "initTransaction" method to initiate a transaction and pop up the widget, It remains on the screen until transaction is completed. The method requires the following parameters:
- key - (String) The Public API key for the business [View Public Key].
- amount - (Double) The amount to charge for the transaction, should be above 10
- currency - (String) The currency of amount for transaction e.g "NGN", "KES". ISO 4217 three-letter code
- phoneNumber - (String) The phone number to which the request will be sent, should begin with the country's dial code without the "+" e.g "234709876543"
- meta - (JSON Object) Object containing any extra information you want recorded with the transaction. Fields within the custom_field object will show up on merchant receipt.
- onSuccess - (Function) It should take a single Object argument which is the transaction object; it is called after a successful transaction
- onFailure - (Function) It should take a single Object argument which is the transaction object; It is called if the transaction fails
- onCancel - (Function) It shouldn’t take any arguments; it is called if the transaction is cancelled while processing transaction.
- channel - (String, optional) the channel to use in sending the request; can be either "ussd" or "whatsapp"; check out get supported channels to get possible channels for the transaction
- chargeCustomer - (Boolean, optional) determines if the customer bears the charges/fees for the transaction; defaults to false
- isTest - (Boolean, optional) determines if this is a test or live transaction; defaults to false (i.e live)
vendyPay.initTransaction({ key: 'test_api_ky_xxxxxxxx', amount: 100, currency: "NGN", phoneNumber: "234709876543", channel: "whatsapp", meta: { "email": "[email protected]", "custom-key": "custom-value" }, chargeCustomer: true, isTest: true, onSuccess: (transaction) => { /* ... */ }, onFailure: (transaction) => { /* ... */ }, onCancel: _ => { /* ... */ } });
Monitoring Transaction State
Get transaction to view the status and details of the transaction and Setup Webhooks to monitor the state of the transaction in real-time which are sent as events. Events are sent when transaction is updated - "transaction_updated", successful - "transaction_success", and fails - "transaction_failed" with the transaction details. A sample webhook response:
{
"event.type": "transaction_success",
"data": {
"amount": 60,
"requestamount": 60,
"delivered": 1,
"vended": 1,
"failed": 0,
"debited": 1,
"processing": 0,
"meta": {
"email": "[email protected]",
"custom-key": "custom-value",
"fee": 0,
"commission": 0,
"charge_customer": false,
"fee_structure": {
"fee": 0,
"percent": true,
"threshold": {
"max": 100,
"min": 0
},
"commission": 0,
"charge_customer": true
},
"total": 60,
"narration": "189c8ff2-18bc-46d5-a2f3-5d78cd2e6e56 PURCHASE 6281400565827151883"
},
"refid": "6281400565827151883",
"vendref": "6281400565827151883",
"debitref": "6281400565827151883",
"currency": "NGN",
"request": "Vendy\nDear Customer, Disu Kayode requested payment of NGN60.\nEnter the amount to pay:",
"msisdn": "2347043556860",
"reason": "189c8ff2-18bc-46d5-a2f3-5d78cd2e6e56 vended successfully",
"raw_reason": "Successful transaction",
"client": "189c8ff2-18bc-46d5-a2f3-5d78cd2e6e56",
"channel": "fcmb",
"method": "ussd",
"account": "5566769145",
"created_on": "2023-05-19 08:22:02",
"updated_on": "2023-05-19 08:22:02",
"vended_date": "2023-05-19 08:22:19",
"debited_date": "2023-05-19 08:22:19",
"page": "pin-page"
}
}